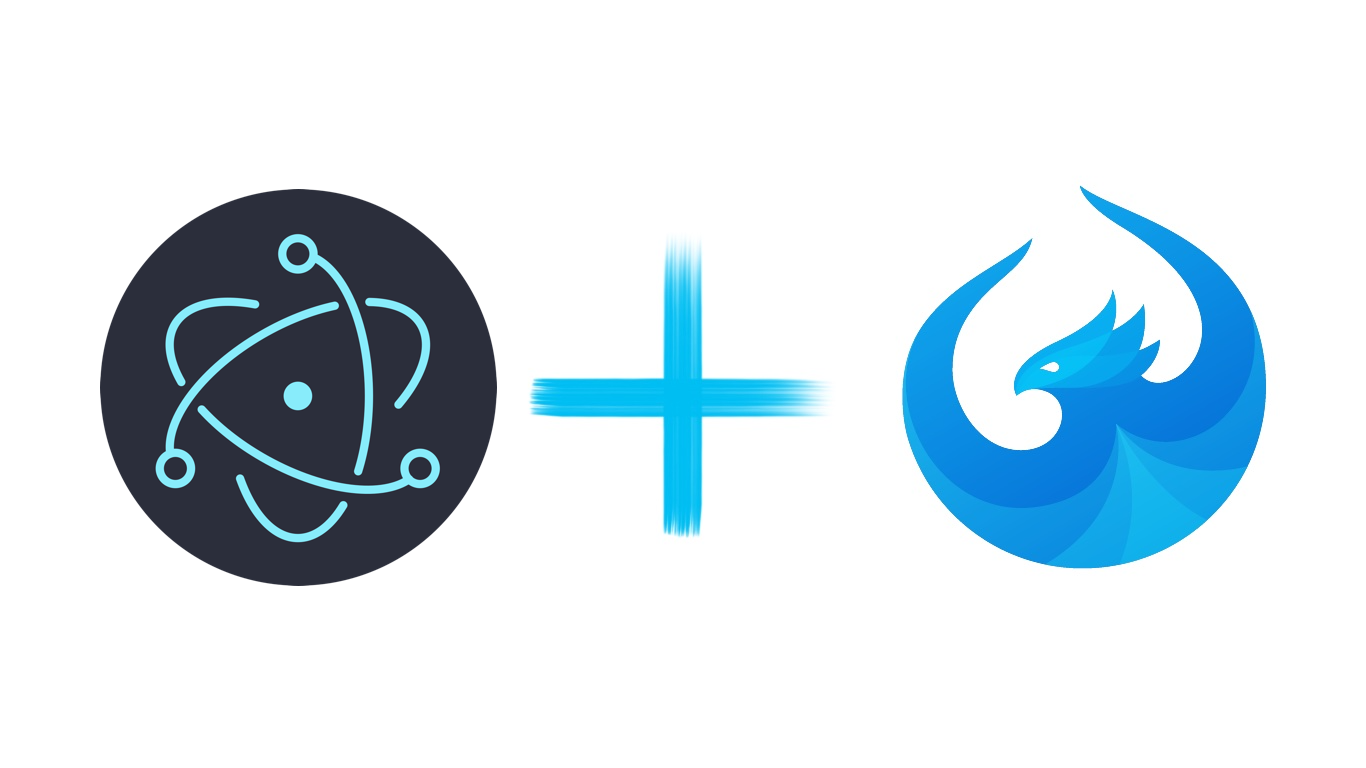
Building desktop applications using UI5 and Electron
Introduction
Recently I've been thinking about building desktop applications, however I'm a JavaScript developer who spends most of his time working with SAP UI5. So what's the best and easiest way to take my skills and knowledge of UI5 JavaScript development and turn that into a deskop application? Electron.
Quick run down on Electron
Electron is a framework for building cross platform desktop apps with JavaScript, HTML, and CSS which so happen to be some of the things I know about! If you're familiar with Cordova think of Electron as the desktop app builder to Cordovas mobile app builder.
Except of course Cordova builds to Windows and Mac and not just IOS/ Android so why did I choose electron?
- I've used Cordova previously (though I've not written a blog on it, maybe I should do this)
- Electron seems to be getting much more frequent and meaningful updates/ improvements
- I'm only interested in building desktop apps
- Because I wanted to try something new.
What are the benefits of Electron?
Why build a desktop application at all? There are a number of different answers to this question but I'll run down in a list some of the most obvious ones to me.
Access to hardware API's
Access to hardware API's is sort of situational, you might not need them but plenty of applications will want to make use of things like battery status or for a newer mac maybe the touchbar?
Offline capability
If I have an application that's setup on the Fiori Launch Pad OR on an internal website and they go down? or there are some internal network issues? I can easily setup some offline capability for the application when access to my API's are down.
Distributable inside of a corporate network
Some clients really just want to deploy an app across the network and have their users use that. It's really much easier telling a user to open up 'x' application rather than goto the browser, goto 'http://myapplication.clientwebsite.com' and go through all those steps for support.
Everyone runs the same build/ application
How many times have you had a defect or bug that only happens in IE8 or some much older version of chrome? You can control the environment and as such you know that when you build and pushout your application (platform potentially pending) that your users should all get exactly the same experience.
Overcoming outdated IE / Chrome version problems
I had a client who controlled/ restricted the use of newer versions of chrome back to Version 33 and we are talking about in 2019!!! Their reasons for running on such an old version of Chrome? They didn't want to break their legacy web apps. Another problem that could be overcome with the use of something like Electron, however there would be a tradeoff of updating/ patching the Electron application for security vulnerabilities.
Getting started
First of all I'm going to assume that you have a nice little UI5 application that you want to actually turn into a desktop application. I'm using a small little quiz application that me and some colleagues made for testing our knowledge on Fiori/ SCP / whatever else we want to add to the quiz. The point being is it's a small app that doesn't currently interact with a DB and so I won't be covering OData/ API connections in this blog post but simply making a working application.
Download and install Node.js
If you haven't already installed node.js do so now from the node website.
Download and run the electron-quick-start app
So not to feel like we're cheating here but why re-invent the wheel? There's a great boilerplate that exists from the electron community and can be simply downloaded, installed and ran using the following commands:
# Clone the Quick Start repository
$ git clone https://github.com/electron/electron-quick-start
# Go into the repository
$ cd electron-quick-start
# Install the dependencies and run
$ npm install && npm start
Then a new electron process should start up and look like this:
Note that your versions of Node, Chromium & Electron will likely differ from mine but here we go! our first electron application up and running! That was really really easy and I can't take any credit for this so far because this is how easy Electron is to get started out of the box.
Understanding our UI5 application and embedding it into our Electron app
My UI5 application is not very special, but I wanted to include a simple screenshot of my folder structure which is quite standard:
Essentially everything lives inside of my 'webapp' folder and as such I'm basically just going to copy and paste this 'webapp' folder into my electron app directory.
Which now means that my electron app directory looks like this:
Note that my editor (VSCode, an Electron app) has even helpfully highlighted this as new files/ folders :)
editing our electron app to run our UI5 application
Inside of our Electron app directory we have a JavaScript file called 'main.js' this file is the first thing that is loaded when we load our electron app up and it does things like create a new browser window, has some boilerplate code for event hooks and stuff like that.
What we need to do is change 1 line from loading index.html (which was the 'hello world' screen we saw before) to using our UI5 index.html which is located inside of our 'webapp' folder.
At the time of writing this change was simply changing
mainWindow.loadFile('index.html')
to this:
mainWindow.loadFile('webapp/index.html')
then, go back to the terminal/ window where we executed npm install && npm start
and close that process with ctrl+c
and simply run npm start
in your terminal.
That's it! you Should then be greeted with your UI5 application running inside of a desktop application as if you were running it on a web server!
^^Mine looks like this, a running MacOS application.
Debugging
Didn't work? You can toggle developer tools right inside of your open electron app and get a look at the console. My UI5 app is using the CDN for it's source right now but what if you were previously using something local to your web server?
Bundling UI5 into our electron app
One of the benefits/ purposes of making a desktop application like this is to eliminate as many of the network calls as possible and make our application potentially work offline if we need. The obvious next step for that is to bundle up a version of UI5 into our app.
To do this we're going to want to download the mobile runtime version of OpenUI5, you might be able to use SAPUI5 in your application subject to licensing restrictions but to make our life easy lets just go with OpenUI5 which can be downloaded from the OpenUI5.org releases section.
Why are we downloading the Mobile runtime when we're making a desktop application? Size. We only need some of the core libraries such as sap.m, sap.ui.layout etc and as such we only need to download the mobile runtime but your case might vary but for the purposes of this tutorial we can download the mobile runtime.
If you want to go ahead and download a different run time that's fine, but be aware that the size of your bundled application will vary in size. You can also choose from different release versions, it just so happens that at the time of writing 1.71.0 was the latest version of OpenUI5 released.
Once downloaded simply extract the zip file and put the 'resources' folder into your electron app folder.
Changing our index.html to use our local version.
Seen as we've added our 'resources' folder to our electron app all we need to do is change our 'src' for our UI5 'sap-ui-core.js' from the CDN src="https://sapui5.hana.ondemand.com/resources/sap-ui-core.js"
or maybe even just src="/resources/sap-ui-core.js"
change this to be src="../resources/sap-ui-core.js"
.
This is because our index.html lives inside of the 'webapp' folder and we need to come out of the 'webapp' folder and into the 'resources' folder.
My project structure and index.html file now look like this, your millage will vary with your index.html file but it should look mostly the same.
Now lets restart our electron service (Ctrl+C
and then npm start
again in our terminal) and we should actually see no difference! other than our app might have loaded quite a bit faster as we're no longer using the CDN to serve our UI5 core.
Building our executable
To make a build for executing on any machine we're going to make use of a package called electron-packager which is a great little package for building out to all of our desired platforms of 'windows, MacOS and Linux' I am going to build for MacOS as I'm using a Mac. To find out all of the relevant arguments for your potential build process please refer to their documentation.
Simply inside of your electron directory run the following command in the terminal:
npm install electron-packager --save-dev
Note there are a number of other build packages around for Electron and they likely have their different uses. This step really is variable depending on your needs but I don't see why electron-packager wouldn't work for most.
Adding our build command
Open up package.json
inside of your editor and you can add a new npm
script command. Do you see where we already have "start": "electron ."
well we can add a new command for our build right here!
As discussed above your build command will likely look quite different to mine, but here's an example of my chosen build command:
"build-mac": "electron-packager . --overwrite --platform=darwin --arch=x64 --prune=true --out=release-builds"
run npm run build-mac
in your terminal or whatever you gave as the 'name' for the script on the left hand side of the key/ value pair.
You should have ended up with something that looks like this:
"scripts": {
"start": "electron .",
"build-mac": "electron-packager . --overwrite --platform=darwin --arch=x64 --prune=true --out=release-builds"
},
This should create a new folder called release-builds
and inside of that folder you'll find your build folder and the application inside.
Building for other platforms
So we want to build for a different platform? Great, just add a new command, how about windows? Lets just take a copy of our build-mac command and make a new command called build-win that might look something like this:
"build-win": "electron-packager . --overwrite --platform=win32 --arch=x64 --prune=true --out=release-builds"
HOWEVER, building for windows didn't actually work for me which is fine because there is documentation to build for windows from a Mac on their github. I could have set this up but decided it was beyond the scope of the blog.
Giving our application a name, description, keywords etc.
To change the name, description, keywords, version number etc associated with our application we're going to have to make an edit to the package.json
file. Simply load up the file and edit the JSON, taking note that the name of an application should be kept to short and use kebab-case.
To give your application display name we'll need to add in a new field of productName which would look like this:
"productName": "Nathan Hand UI5 Quiz",
This is mostly just changing values in the package.json
so feel free to update them as much or as little as you want.
Conclusion
There we have it, a built up version of a UI5 application as an executable and it only took us probably 30? minutes if that. There are obviously lots of places to divulge from this post as you will likely want to setup a build pipeline or build out for multiple platforms in a robust fashion but ultimately we went from having to deploy our application to a website or the Fiori Launchpad to potentially just allowing our users to download our application or we could have added it to a store and allow for downloads that way the possibilities are endless.
Want a follow up series? or have any questions? Let me know in the comments or reach out on Twitter.
···