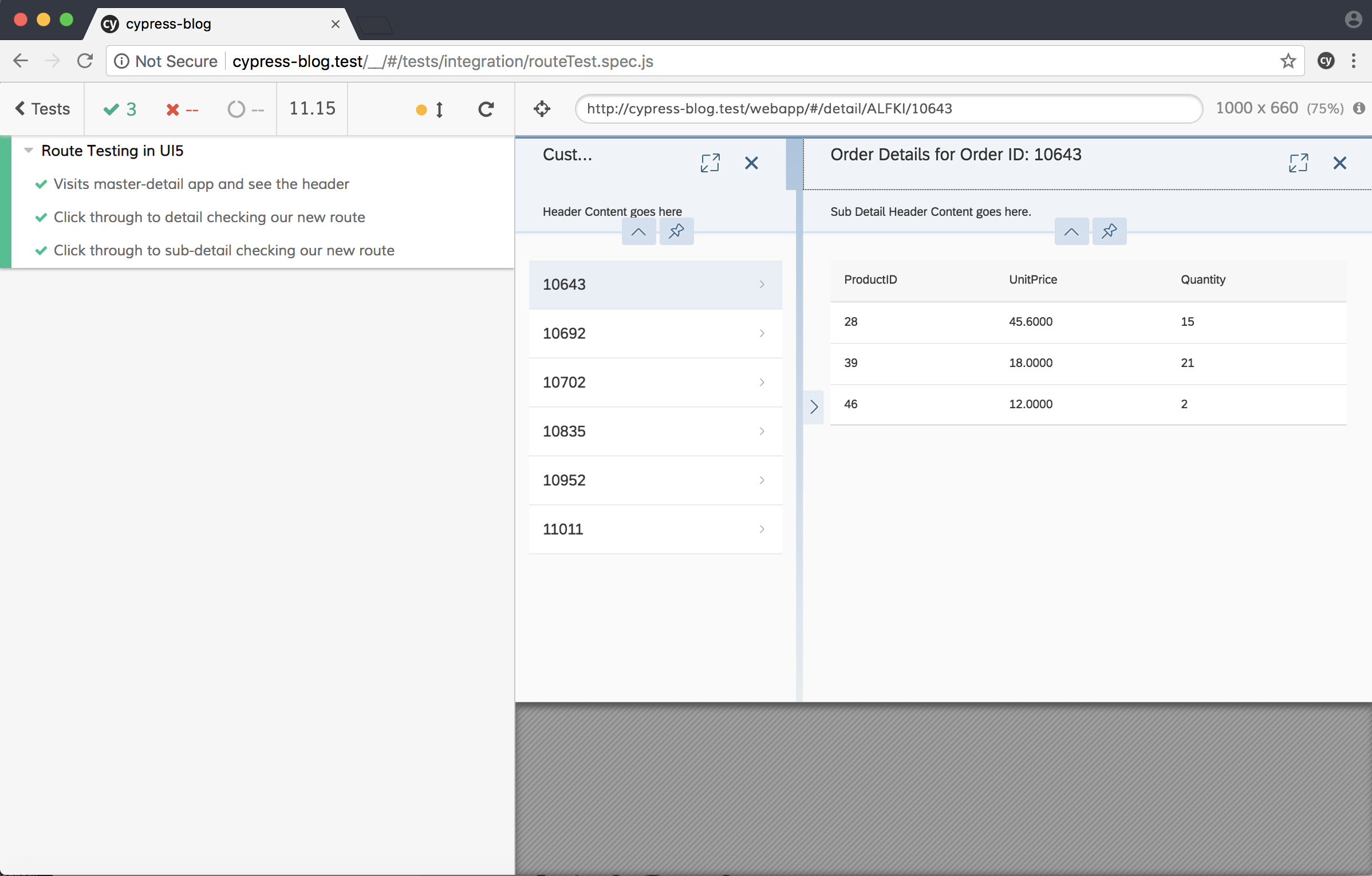
getting started with cypress and ui5
This is a quick tutorial about setting up Cypress to write some automated tests for use with UI5.
I should start this blog with quite a shameful statement, one that I think many of my fellow UI5 developers are also guilty of for one reason or another.
I haven't written a single test using the preferred UI5 testing frameworks, be it QUnit or OPA5.
So knowing that I know absolutely nothing about testing applications in UI5 I decided to look into it. I could have gone towards QUnit and OPA5 and I likely still will look into those in the near future. However, I had recently heard about Cypress and decided that It looked powerful, easy and has quite a few nice features.
Things you'll need
Install ExpressUI5 and create a master-detail project (optional)
If you already have a project where you want to test out Cypress then go ahead and skip to "install Cypress on your project" otherwise follow the below steps.
I have recently had some time to get around to making some more templates for my template package ExpressUI5. This package is simply a collection of small UI5 applications that I've made and can quickly generate a project with your own project name & namespace which is perfect for playing around/ making a POC. see more in my blog
Run the following commands to get up and running with your new master-detail application:
npm install -g expressui5
cd /your/development/folder
expressui5
follow the on-screen prompts selecting the template "master-detail-flexible-columns" and filling in your project name and project namespace accordingly.
This template at the time of writing isn't perfect/ complete but I've deployed it regardless as it's really only missing some cleanup.
Run the application on your local web server, it should be setup with data from Northwind and you should see some data like this:
Install Cypress on your project
I suggest checking out the Cypress installation guide on how to install Cypress to your project but for those familiar with NPM I would simply run the following in your project folder:
npm init (only do this if you've not setup/ used NPM in this project so far)
npm install cypress --save-dev
This will then download and install Cypress into your project folder and it will automatically create a number of example tests for you.
Open up your favorite editor and you should now see a new folder called "cypress", it should look something like this:
We mostly only care about the folder called "integration" for now as that's where all the tests for this application will sit.
Inside of the integration folder create a new file, we're going to focus the tests for this application around the routing and subsequent data binding requests that happen as a result of hitting our routes and so I've setup my file as the following:
routeTest.spec.js (new file)
describe('Route Testing in UI5', function() {
it('Visits master-detail app and see the header', function() {
cy.visit('http://cypress-blog.test/webapp/') //change according to your webserver
cy.contains('Master Header').should('be.visible') //change text inside of contains relevant to some text seen in your applcation.
})
})
It's important to note that you will need to change the URL for cy.visit to match the URL of your application, I use a local webserver called Valet which uses DnsMasq which proxies all of my requests to *.test domain to my local file system but you might have something simpler like "http://localhost:8080/yourApplicationDirectory/webapp/".
Launching Cypress
Now open up Cypress from the command line/ terminal as described in the documentation but the easiest way that I found was through the following command:
npx cypress open
This will launch a new application which should look something like this:
Now if we click on our new file that we just created this will launch a new Google chrome window and will automatically begin running our tests which should output like the following:
Congratulations! we've ran our first passing test using Cypress for UI5!
More tests!
We want to test our routing inside of our UI5 application, so to do this with a master-detail application we need to make use of some ODATA. The application I have is setup to use Northwind (a public ODATA service provided by Microsoft) so I'm going to use examples in my tests that look for data that I'm aware of coming from Northwind.
Clicking through to our detail page test
it('Click through to detail checking our new route', function() {
cy.server()
cy.route('https://cors-anywhere.herokuapp.com/http://services.odata.org/V2/Northwind/Northwind.svc/$metadata?sap-language=EN').as('metadata')
cy.route('https://cors-anywhere.herokuapp.com/http://services.odata.org/V2/Northwind/Northwind.svc/Customers?$skip=0&$top=20').as('customerRequest')
cy.visit('http://cypress-blog.test/webapp/')
cy.wait('@metadata')
cy.wait('@customerRequest')
cy.contains('Maria').click()
cy.url().should('include', '/detail/ALFKI')
})
The above test has a few different things going on, at the top of the test we define 2 routes/ XHR requests that we're going to look out for. These two requests are our metadata request (triggered first, and needs to complete before our other request will take place) and our customerRequest request which returns a list of customers.
In our test we once again visit the baseURL of our application and then we wait for both our metadata and customerRequest call to complete before the rest of the test can continue. (indicated by cy.wait)
Then once loaded I know that Northwind will return the data of "Maria" and I can instruct Cypress to click Maria which will then kick off the navigation to my detail page which we check for in my final line to see the URL change which completes our test.
Clicking through to our sub-detail page/ end column
it('Click through to sub-detail checking our new route', function() {
cy.server()
cy.route('https://cors-anywhere.herokuapp.com/http://services.odata.org/V2/Northwind/Northwind.svc/$metadata?sap-language=EN').as('metadata')
cy.route('https://cors-anywhere.herokuapp.com/http://services.odata.org/V2/Northwind/Northwind.svc/Customers(\'ALFKI\')?$expand=Orders').as('orderRequest')
cy.visit('http://cypress-blog.test/webapp/#/detail/ALFKI')
cy.wait('@metadata')
cy.wait('@orderRequest')
cy.contains('10643').click()
cy.url().should('include', '/detail/ALFKI/10643')
})
Most of the above is exactly the same as our previous test, but we're going to the next level/ end column of our master-detail application. We skip part of the previous tests checks/ waiting around because we instead visit our detail page directly but otherwise it's pretty much the same test but on the last level.
Conclusion
We have 3 tests all of which hit our routes so we know that nothing has gone horribly wrong with our application! Now It's quite obvious that the fact that all 3 tests took about 11 seconds (I've had much worse from Northwind) to run isn't exactly ideal, however that's mostly down to the Northwind data service used and so would be quicker in real world examples.
Running tests in parallel is also something that you can do/ can setup with some time so I don't really think that tests will take too long to run in the long term/ a proper setup.
I hope that this has been a quick and easy introduction to using Cypress with UI5 and how easily you can get into automated testing. I think that Cypress is an excellent tool and it's only in Beta at the time of writing but I can already see it becoming a popular testing tool among projects and teams alike.
Questions? Comments or Concerns? Let me known down below in the comments or on my Twitter.
···